Start Date
Number of days to add or subtract
Add years, months or weeks
Steps to use the Date Calculator Tool
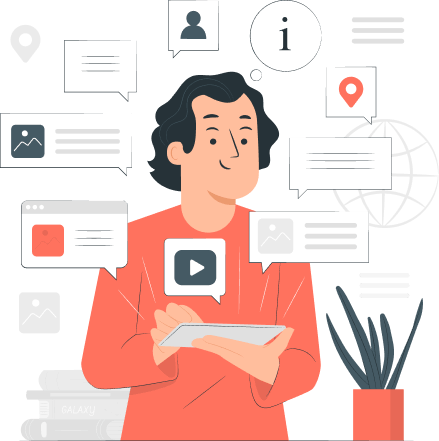
- Select the 'Start Date' to which you want to add the days. Click the date picker or edit the default date (mm/dd/yyyy format)
- Enter the number of days in the Number of days field.
- You can add weeks, months and number of years by entering the value in the respective fields
- Click on "Calculate" button to find the end date
- To subtract days, weeks, months or years, enter the value and select 'Subtract' option and then click 'Calculate' to find the subtract from the given date
- If you want to find the date based on work days or business days from a given date, then enable the option 'Work Days / Business Days'. Work Days is taken as 5 days / 40 hours per week
Number of Days from Today
Calendar Days from Today (Friday, 13 Jun 2025) | Date |
---|---|
3 days from today | Monday, 16 Jun 2025 |
4 days from today | Tuesday, 17 Jun 2025 |
5 days from today | Wednesday, 18 Jun 2025 |
7 days from today | Friday, 20 Jun 2025 |
10 days from today | Monday, 23 Jun 2025 |
14 days from today | Friday, 27 Jun 2025 |
15 days from today | Saturday, 28 Jun 2025 |
20 days from today | Thursday, 3 Jul 2025 |
21 days from today | Friday, 4 Jul 2025 |
28 days from today | Friday, 11 Jul 2025 |
30 days from today | Sunday, 13 Jul 2025 |
31 days from today | Monday, 14 Jul 2025 |
35 days from today | Friday, 18 Jul 2025 |
40 days from today | Wednesday, 23 Jul 2025 |
42 days from today | Friday, 25 Jul 2025 |
50 days from today | Saturday, 2 Aug 2025 |
56 days from today | Friday, 8 Aug 2025 |
60 days from today | Tuesday, 12 Aug 2025 |
75 days from today | Wednesday, 27 Aug 2025 |
80 days from today | Monday, 1 Sep 2025 |
90 days from today | Thursday, 11 Sep 2025 |
100 days from today | Sunday, 21 Sep 2025 |
120 days from today | Saturday, 11 Oct 2025 |
150 days from today | Monday, 10 Nov 2025 |
180 days from today | Wednesday, 10 Dec 2025 |
200 days from today | Tuesday, 30 Dec 2025 |
250 days from today | Wednesday, 18 Feb 2026 |
300 days from today | Thursday, 9 Apr 2026 |
360 days from today | Monday, 8 Jun 2026 |
400 days from today | Saturday, 18 Jul 2026 |
500 days from today | Monday, 26 Oct 2026 |
730 days from today | Sunday, 13 Jun 2027 |
1000 days from today | Thursday, 9 Mar 2028 |
1095 days from today | Monday, 12 Jun 2028 |
Number of Work Days from Today
Business Days from Today (Friday, 13 Jun 2025) | Date |
---|---|
1 business days from today | Monday, 16 Jun 2025 |
2 business days from today | Tuesday, 17 Jun 2025 |
3 business days from today | Wednesday, 18 Jun 2025 |
4 business days from today | Thursday, 19 Jun 2025 |
5 business days from today | Friday, 20 Jun 2025 |
6 business days from today | Monday, 23 Jun 2025 |
7 business days from today | Tuesday, 24 Jun 2025 |
8 business days from today | Wednesday, 25 Jun 2025 |
9 business days from today | Thursday, 26 Jun 2025 |
10 business days from today | Friday, 27 Jun 2025 |
12 business days from today | Tuesday, 1 Jul 2025 |
14 business days from today | Thursday, 3 Jul 2025 |
15 business days from today | Friday, 4 Jul 2025 |
20 business days from today | Friday, 11 Jul 2025 |
21 business days from today | Monday, 14 Jul 2025 |
28 business days from today | Wednesday, 23 Jul 2025 |
30 business days from today | Friday, 25 Jul 2025 |
31 business days from today | Monday, 28 Jul 2025 |
35 business days from today | Friday, 1 Aug 2025 |
40 business days from today | Friday, 8 Aug 2025 |
42 business days from today | Tuesday, 12 Aug 2025 |
45 business days from today | Friday, 15 Aug 2025 |
50 business days from today | Friday, 22 Aug 2025 |
56 business days from today | Monday, 1 Sep 2025 |
60 business days from today | Friday, 5 Sep 2025 |
75 business days from today | Friday, 26 Sep 2025 |
80 business days from today | Friday, 3 Oct 2025 |
90 business days from today | Friday, 17 Oct 2025 |
100 business days from today | Friday, 31 Oct 2025 |
120 business days from today | Friday, 28 Nov 2025 |
150 business days from today | Friday, 9 Jan 2026 |
180 business days from today | Friday, 20 Feb 2026 |
360 business days from today | Friday, 30 Oct 2026 |
Add Date Calculator
How to add Calendar Days to a date using Javascript?
To Add days to a given date
Create date with given start date in string format start Date = new Date(start date in string);
0 means same day, -1 previous day, +32 goes into next month and adds remaining days of that month.
To subtract, pass -ve value and the days will be computed
The return value of the set Date is time in milliseconds format
Convert the timestamp to readable text format
Add Business Days / Add Work Days Calculator
How to add work days or business days to a date?
Find number of weeks - assuming 5 business days, find the number of weeks
Number of days / 5 = weeks
Take whole part for weeks and remainder part for days
Number of days % 5 = remainder days
week count = truncate (number of days / 5);
day count= number of days % 5;
Multiply by 7 to get actual calendar days use that to add to the start date
days to add = weekCount * 7 + dayCount;
Get the day information. If it is 6 (friday), then add 2 to the number of days
If it is 0 (Sunday), then add 1 to the number of days